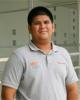
จากนั้นเริ่มเขียน Code กันเลยครับ โดยทำตามขั้นตอนดังนี้
1) เปิด Eclipse ขึ้นมา ไปที่ File – New – Android Project และตั้งชื่อ Project ดังรูป
2) เลือก SDK
3)ตั้งชื่อ Package Name
4)แก้ไขไฟล์ /res/layout/main.xml ดังนี้
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
02 |
< LinearLayout xmlns:android = "http://schemas.android.com/apk/res/android" |
03 |
android:layout_width = "fill_parent" |
04 |
android:layout_height = "fill_parent" |
05 |
android:orientation = "vertical" > |
06 |
< TextView |
07 |
android:layout_width = "fill_parent" |
08 |
android:layout_height = "wrap_content" |
09 |
android:text = "Connect To Mysql Example" /> |
10 |
< EditText |
11 |
android:id = "@+id/txt_hn" |
12 |
android:layout_width = "match_parent" |
13 |
android:layout_height = "wrap_content" |
14 |
android:hint = "HN" > |
15 |
</ EditText > |
16 |
< EditText |
17 |
android:id = "@+id/txt_name" |
18 |
android:layout_width = "match_parent" |
19 |
android:layout_height = "wrap_content" |
20 |
android:hint = "Name-Lastname" /> |
21 |
< EditText |
22 |
android:id = "@+id/txt_age" |
23 |
android:layout_width = "match_parent" |
24 |
android:layout_height = "wrap_content" |
25 |
android:hint = "Age" /> |
26 |
< LinearLayout |
27 |
android:layout_width = "match_parent" |
28 |
android:layout_height = "wrap_content" |
29 |
android:orientation = "horizontal" > |
30 |
< Button |
31 |
android:id = "@+id/btn_insert" |
32 |
android:layout_width = "wrap_content" |
33 |
android:layout_height = "wrap_content" |
34 |
android:text = "Insert" /> |
35 |
< Button |
36 |
android:id = "@+id/btn_select" |
37 |
android:layout_width = "wrap_content" |
38 |
android:layout_height = "wrap_content" |
39 |
android:text = "Select" /> |
40 |
< Button |
41 |
android:id = "@+id/btn_update" |
42 |
android:layout_width = "wrap_content" |
43 |
android:layout_height = "wrap_content" |
44 |
android:text = "Update" /> |
45 |
< Button |
46 |
android:id = "@+id/btn_delete" |
47 |
android:layout_width = "wrap_content" |
48 |
android:layout_height = "wrap_content" |
49 |
android:text = "Delete" /> |
50 |
</ LinearLayout > |
51 |
< ScrollView |
52 |
android:id = "@+id/scrollView1" |
53 |
android:layout_width = "match_parent" |
54 |
android:layout_height = "wrap_content" > |
55 |
< LinearLayout |
56 |
android:layout_width = "match_parent" |
57 |
android:layout_height = "match_parent" > |
58 |
< TextView |
59 |
android:id = "@+id/tv_res" |
60 |
android:layout_width = "match_parent" |
61 |
android:layout_height = "wrap_content" |
62 |
android:text = "" /> |
63 |
</ LinearLayout > |
64 |
</ ScrollView > |
65 |
</ LinearLayout > |
import java.io.BufferedReader;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.ArrayList;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.message.BasicNameValuePair;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import android.app.Activity;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
Button btn_insert;
Button btn_update;
Button btn_delete;
EditText txt_hn;
EditText txt_name;
EditText txt_age;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
// ตั้งค่าตัวแปล View
tv_res = (TextView)findViewById(R.id.tv_res);
txt_hn = (EditText)findViewById(R.id.txt_hn);
txt_name = (EditText)findViewById(R.id.txt_name);
txt_age = (EditText)findViewById(R.id.txt_age);
btn_select.setOnClickListener(this);
btn_insert.setOnClickListener(this);
btn_update.setOnClickListener(this);
btn_delete.setOnClickListener(this);
public void onClick(View v){
switch(v.getId()){
case R.id.btn_select:
{
select();
break;
}
case R.id.btn_insert:
{
insert();
break;
}
case R.id.btn_update:
{
update();
break;
}
case R.id.btn_delete:
{
delete();
break;
}
}
}
txt_hn.setText("");
txt_name.setText("");
txt_age.setText("");
}
try{
String hn = txt_hn.getText().toString().trim();
String name = txt_name.getText().toString().trim();
String age = txt_age.getText().toString().trim();
if ( hn.equals("") || name.equals("") || age.equals("") ){
return ;
}
ArrayList<NameValuePair> nameValuePairs = new ArrayList<NameValuePair>();
nameValuePairs.add(new BasicNameValuePair("isAdd","true"));
nameValuePairs.add(new BasicNameValuePair("hn",hn));
nameValuePairs.add(new BasicNameValuePair("name",name));
nameValuePairs.add(new BasicNameValuePair("age",age));
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://10.0.2.2:82/php_set_data.php");//Change IP to you WebServer
httppost.setEntity(new UrlEncodedFormEntity(nameValuePairs,"UTF-8"));
httpclient.execute(httppost);
clsText();
}catch(Exception e){
Log.d("log_err", "Error in http connection " + e.toString());
}
}
// Your update algorithm
Toast.makeText(getApplicationContext(), "update",Toast.LENGTH_SHORT).show();
}
// Your delete algorithm
Toast.makeText(getApplicationContext(), "delete",Toast.LENGTH_SHORT).show();
}
tv_res.setText("");
InputStream is = null;
String js_result = "";
try {
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://10.0.2.2:82/php_get_data.php");
HttpResponse response = httpclient.execute(httppost);
HttpEntity entity = response.getEntity();
is = entity.getContent();
} catch (Exception e) {
Log.d("log_err", "Error in http connection " + e.toString());
}
try {
BufferedReader reader = new BufferedReader(new InputStreamReader(is,"UTF-8"));
StringBuilder sb = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
sb.append(line);
}
is.close();
js_result = sb.toString();
} catch (Exception e) {
Log.e("log_tag", "Error converting result " + e.toString());
}
try {
final JSONArray jArray = new JSONArray(js_result);
for (int i = 0; i < jArray.length(); i++) {
JSONObject jo = jArray.getJSONObject(i);
String hn = jo.getString("hn");
String name = jo.getString("name");
String age = String.valueOf(jo.getInt("age"));
String date_serv = jo.getString("date_serv");
Log.d("log",hn+","+name+","+age+","+date_serv);
tv_res.append(hn+","+name+","+age+","+date_serv+"\n");
}
} catch (JSONException e) {
Log.e("log_tag", "Error parsing data " + e.toString());
}
}
}
6)แก้ไขไฟล์ AndroidManifest.xml ดังนี้
01 |
<? xml version = "1.0" encoding = "utf-8" ?> |
02 |
< manifest xmlns:android = "http://schemas.android.com/apk/res/android" |
03 |
package = "com.Example" |
04 |
android:versionCode = "1" |
05 |
android:versionName = "1.0" > |
06 |
< uses-sdk android:minSdkVersion = "10" /> |
07 |
< uses-permission android:name = "android.permission.INTERNET" /> |
08 |
< application |
09 |
android:icon = "@drawable/ic_launcher" |
10 |
android:label = "@string/app_name" > |
11 |
< activity |
12 |
android:name = ".Main" |
13 |
android:label = "@string/app_name" > |
14 |
< intent-filter > |
15 |
< action android:name = "android.intent.action.MAIN" /> |
16 |
< category android:name = "android.intent.category.LAUNCHER" /> |
17 |
</ intent-filter > |
18 |
</ activity > |
19 |
</ application > |
20 |
</ manifest > |
ซึ่งตอนนี้เราได้สร้าง Application ฝั่ง Android เรียบร้อยแล้ว
ขั้นตอนต่อไปเราต้องไปสร้าง Application ฝั่ง Server ก็คือ ไฟล์ PHP ของเรานั่นเองครับ
ในบทความนี้ผมสร้าง PHP ขึ้นมา 2 ไฟล์ คือ
- ไฟล์ php_set_data.php ทำหน้าที่ insert
01 |
<?php |
02 |
header( "content-type:text/javascript;charset=utf-8" ); |
03 |
$con =mysql_connect( 'localhost' , 'sa' , 'sa' ) or die (mysql_error()); // เปลี่ยน localhost เป็น ip ของ mysql server |
04 |
mysql_select_db( 'android' ) or die (mysql_error()); |
05 |
mysql_query( "SET NAMES UTF8" ); |
06 |
if (isset( $_POST )){ |
07 |
if ( $_POST [ 'isAdd' ]== 'true' ){ |
08 |
$hn = $_POST [ 'hn' ]; |
09 |
$name = $_POST [ 'name' ]; |
10 |
$age = $_POST [ 'age' ]; |
11 |
$date = date ( 'Y-m-d' ); |
12 |
$sql = "INSERT INTO `patient` (`hn`, `name`, `age`, `date_serv`) VALUES ('$hn', '$name', '$age', '$date')" ; |
13 |
mysql_query( $sql ); |
14 |
} |
15 |
} |
16 |
mysql_close(); |
17 |
?> |
-ไฟล์ php_get_data.php ทำหน้าที่ select
01 |
<?php |
02 |
header( "content-type:text/javascript;charset=utf-8" ); |
03 |
$con =mysql_connect( 'localhost' , 'sa' , 'sa' ) or die (mysql_error()); // เปลี่ยน localhost เป็น ip ของ mysql server |
04 |
mysql_select_db( 'android' ) or die (mysql_error()); |
05 |
mysql_query( "SET NAMES UTF8" ); |
06 |
$sql = "SELECT * FROM patient" ; |
07 |
$res =mysql_query( $sql ); |
08 |
while ( $row =mysql_fetch_assoc( $res )){ |
09 |
$output []= $row ; |
10 |
} |
11 |
print (json_encode( $output )); |
12 |
mysql_close(); |
13 |
?> |
จากนั้นไปที่ Mysql Server ให้ท่านสร้าง database ชื่อ android ขึ้นมา
และให้ create table ชื่อ patient ด้วยคำสั่งดังนี้
01 |
DROP TABLE IF EXISTS `patient`; |
02 |
CREATE TABLE `patient` ( |
03 |
`hn` varchar (9) DEFAULT '' , |
04 |
` name ` varchar (100) DEFAULT NULL , |
05 |
`age` int (11) DEFAULT NULL , |
06 |
`date_serv` varchar (80) DEFAULT '0000-00-00' |
07 |
) ENGINE=InnoDB DEFAULT CHARSET=tis620; |
08 |
09 |
-- ---------------------------- |
10 |
-- ทดสอบใส่ข้อมูล 2 row |
11 |
-- ---------------------------- |
12 |
INSERT INTO `patient` VALUES ( '333' , 'กกกกก' , '12' , '0000-00-00' ); |
13 |
INSERT INTO `patient` VALUES ( '222' , 'aasss' , '45' , '0000-00-00' ); |
เมื่อเสร็จสิ้นขั้นตอนทางฝั่ง Server แล้ว กลับไปที่ Eclipse แล้วลองรันโปรเจค TestMySql ดูครับ
Credit & SourceCode : http://android4health.wordpress.com/2012/06/25/android_con_mysql/