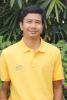

Registration Page
Let’s assume, below is our registration page, it contains username input field, and span HTML element with ID user-result to dump ajax results:
Code |
<div id="registration-form"> <label for="username">Enter Username : <input name="username" type="text" id="username" maxlength="15"> <span id="user-result"></span> </label> </div> |
With help of jQuery, we can make Ajax calls to PHP page when the user releases a key on the keyboard. Method used here is .keyup().
Code |
$("#username").keyup(function (e) { //do some stuff }); |
To make Ajax calls, we will be using jQuery $.post method, it is the easiest way to load data from the server using a HTTP POST request, it also lets us send some additional data along, which is perfect for sending username POST variable. So heres actual jQuery code to send username POST variable from input field to another page.
Code |
$("#username").keyup(function (e) { //user types username on inputfiled var username = $(this).val(); //get the string typed by user $.post('check_username.php', {'username':username}, function(data) { //make ajax call to check_username.php $("#user-result").html(data); //dump the data received from PHP page }); }); |
Return Username Availability
Our registration page makes Ajax calls to PHP file to check username availability. Heres our simple PHP page check_username.php, it returns enough data from the database we need for the registration process.Code |
if(isset($_POST["username"])) { //connect to database $connecDB = mysqli_connect(HOST, USERNAME, PASS, DBNAME)or die('could not connect to database'); //received username value from registration page $username = $_POST["username"]; //check username in db $results = mysqli_query($connecDB,"SELECT id FROM username_list WHERE username='$username'"); $username_exist = mysqli_num_rows($results); //records count //if returned value is more than 0, username is not available if($username_exist) { die('<img src="imgs/not-available.png" />'); }else{ die('<img src="imgs/available.png" />'); } } |
Heres the complete code for check_username.php, as it is dangerous to use input data directly into MySQL queries, it is always a good idea to have them sanitized and use some extra security. I have sanitized POST value with PHP filter_var below and checked whether incoming is really Ajax request, using PHP $_SERVER['HTTP_X_REQUESTED_WITH'].
Code |
###### db ########## $db_username = 'xxxx'; $db_password = 'xxxx'; $db_name = 'xxxx'; $db_host = 'localhost'; ################ //check we have username post var if(isset($_POST["username"])) { //check if its an ajax request, exit if not if(!isset($_SERVER['HTTP_X_REQUESTED_WITH']) AND strtolower($_SERVER['HTTP_X_REQUESTED_WITH']) != 'xmlhttprequest') { die(); } //try connect to db $connecDB = mysqli_connect($db_host, $db_username, $db_password,$db_name)or die('could not connect to database'); //trim and lowercase username $username = strtolower(trim($_POST["username"])); //sanitize username $username = filter_var($username, FILTER_SANITIZE_STRING, FILTER_FLAG_STRIP_LOW|FILTER_FLAG_STRIP_HIGH); //check username in db $results = mysqli_query($connecDB,"SELECT id FROM username_list WHERE username='$username'"); //return total count $username_exist = mysqli_num_rows($results); //total records //if value is more than 0, username is not available if($username_exist) { echo '<img src="imgs/not-available.png" />'; }else{ echo '<img src="imgs/available.png" />'; } //close db connection mysqli_close($connecDB); } |
จาก : http://www.sanwebe.com/2013/04/username-live-check-using-ajax-php